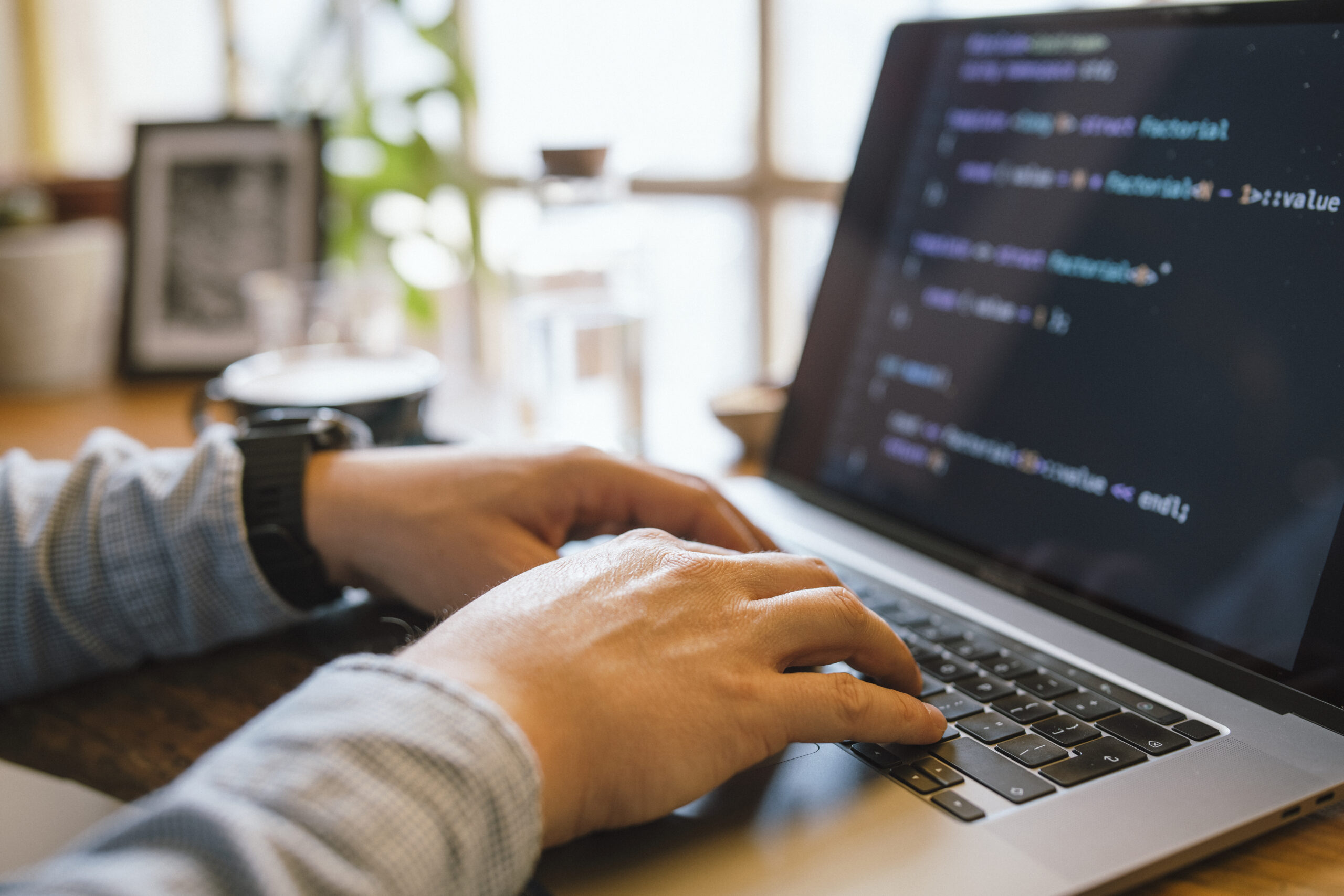
Debugging is Probably the most important — nevertheless normally overlooked — abilities within a developer’s toolkit. It's actually not almost correcting damaged code; it’s about being familiar with how and why things go wrong, and Studying to Believe methodically to solve issues effectively. No matter whether you're a novice or possibly a seasoned developer, sharpening your debugging capabilities can preserve hrs of disappointment and drastically boost your productivity. Listed here are a number of methods to help builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Resources
One of the fastest strategies developers can elevate their debugging expertise is by mastering the tools they use every day. When producing code is a single A part of development, recognizing tips on how to communicate with it successfully all through execution is equally vital. Present day improvement environments occur Outfitted with potent debugging abilities — but a lot of developers only scratch the area of what these instruments can do.
Get, for instance, an Built-in Growth Atmosphere (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools help you set breakpoints, inspect the worth of variables at runtime, phase by means of code line by line, and even modify code to the fly. When utilized the right way, they Allow you to notice specifically how your code behaves throughout execution, which is priceless for tracking down elusive bugs.
Browser developer equipment, such as Chrome DevTools, are indispensable for entrance-finish builders. They permit you to inspect the DOM, observe community requests, see authentic-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and community tabs can transform aggravating UI difficulties into manageable jobs.
For backend or system-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB provide deep Management around operating processes and memory administration. Discovering these resources could have a steeper Mastering curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, come to be comfortable with Edition Management devices like Git to be familiar with code history, locate the exact minute bugs ended up released, and isolate problematic variations.
Ultimately, mastering your tools indicates likely further than default settings and shortcuts — it’s about developing an intimate knowledge of your improvement surroundings in order that when troubles come up, you’re not dropped at nighttime. The higher you recognize your equipment, the more time you'll be able to devote fixing the actual issue instead of fumbling via the process.
Reproduce the Problem
One of the most critical — and infrequently forgotten — methods in powerful debugging is reproducing the trouble. Prior to jumping into your code or building guesses, developers require to produce a reliable setting or situation exactly where the bug reliably seems. Devoid of reproducibility, repairing a bug becomes a activity of probability, typically leading to squandered time and fragile code adjustments.
The first step in reproducing a dilemma is collecting as much context as is possible. Request questions like: What steps resulted in The difficulty? Which surroundings was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it gets to be to isolate the precise circumstances less than which the bug happens.
As you’ve collected more than enough facts, make an effort to recreate the problem in your neighborhood environment. This might necessarily mean inputting precisely the same data, simulating equivalent person interactions, or mimicking program states. If The difficulty appears intermittently, contemplate crafting automated assessments that replicate the sting instances or condition transitions associated. These exams not simply help expose the trouble but will also stop regressions Sooner or later.
In some cases, the issue could possibly be ecosystem-particular — it would transpire only on certain working programs, browsers, or less than particular configurations. Making use of equipment like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a step — it’s a state of mind. It calls for endurance, observation, and also a methodical solution. But once you can regularly recreate the bug, you are previously halfway to repairing it. By using a reproducible circumstance, You should utilize your debugging applications extra effectively, test potential fixes safely, and communicate more Evidently with your team or users. It turns an summary criticism right into a concrete problem — and that’s exactly where developers prosper.
Study and Comprehend the Error Messages
Mistake messages will often be the most beneficial clues a developer has when a thing goes Erroneous. In lieu of observing them as aggravating interruptions, developers should master to take care of error messages as direct communications within the process. They typically let you know precisely what transpired, wherever it occurred, and occasionally even why it transpired — if you understand how to interpret them.
Commence by studying the information meticulously and in comprehensive. A lot of developers, specially when beneath time pressure, look at the initial line and immediately get started generating assumptions. But deeper from the error stack or logs may perhaps lie the real root trigger. Don’t just duplicate and paste error messages into search engines — examine and comprehend them to start with.
Split the mistake down into components. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it point to a certain file and line number? What module or operate brought on it? These queries can guideline your investigation and level you towards the responsible code.
It’s also valuable to understand the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java normally adhere to predictable designs, and learning to recognize these can considerably speed up your debugging approach.
Some faults are vague or generic, and in All those situations, it’s very important to examine the context during which the mistake happened. Check connected log entries, enter values, and up to date changes inside the codebase.
Don’t forget compiler or linter warnings possibly. These frequently precede greater difficulties and supply hints about potential bugs.
In the end, error messages will not be your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, assisting you pinpoint troubles speedier, cut down debugging time, and turn into a extra efficient and confident developer.
Use Logging Properly
Logging is Just about the most strong instruments in a very developer’s debugging toolkit. When made use of effectively, it offers serious-time insights into how an software behaves, encouraging you understand what’s going on underneath the hood without having to pause execution or move in the code line by line.
A very good logging system starts off with figuring out what to log and at what stage. Widespread logging stages incorporate DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for specific diagnostic facts through growth, Data for basic occasions (like successful get started-ups), Alert for likely concerns that don’t break the applying, Mistake for real problems, and Lethal if the method can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure vital messages and decelerate your method. Focus on critical functions, state improvements, input/output values, and important determination points in the code.
Structure your log messages Obviously and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
During debugging, logs Allow you to keep track of how variables evolve, what problems are met, and what branches of logic are executed—all with no halting This system. They’re Specifically important in creation environments where by stepping by means of code isn’t probable.
Furthermore, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Finally, sensible logging is about harmony and clarity. With a properly-assumed-out logging method, you may reduce the time it will take to identify challenges, achieve further visibility into your programs, and Enhance the In general maintainability and reliability of your respective code.
Think Just like a Detective
Debugging is not merely a technical activity—it is a form of investigation. To efficiently detect and repair bugs, developers have to solution the process like a detective fixing a thriller. This way of thinking helps break down complicated concerns into manageable areas and observe clues logically to uncover the foundation induce.
Start by gathering evidence. Look at the signs and symptoms of the trouble: error messages, incorrect output, or functionality difficulties. The same as a detective surveys against the law scene, accumulate just as much suitable facts as you could without the need of leaping to conclusions. Use logs, take a look at scenarios, and person stories to piece jointly a clear image of what’s taking place.
Subsequent, form hypotheses. Ask yourself: What can be creating this behavior? Have any changes a short while ago been built to your codebase? Has this situation occurred ahead of beneath comparable instances? The intention is to narrow down possibilities and detect opportunity culprits.
Then, exam your theories systematically. Endeavor to recreate the situation in a very managed surroundings. Should you suspect a selected operate or component, isolate it and validate if The problem persists. Just like a detective conducting interviews, inquire your code questions and Permit the outcomes guide you nearer to the truth.
Pay back near interest to compact information. Bugs frequently disguise while in the least predicted locations—similar to a missing semicolon, an off-by-a person error, or a race affliction. Be comprehensive and affected individual, resisting the urge to patch The problem without thoroughly comprehending it. Momentary fixes could hide the true problem, only for it to resurface afterwards.
And read more finally, keep notes on Whatever you tried and uncovered. Equally as detectives log their investigations, documenting your debugging method can help save time for long term difficulties and help Some others understand your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical capabilities, solution difficulties methodically, and come to be more effective at uncovering concealed issues in sophisticated devices.
Write Exams
Producing checks is one of the best solutions to improve your debugging abilities and Total enhancement performance. Tests not just support capture bugs early and also function a security Web that gives you self-confidence when generating alterations towards your codebase. A perfectly-tested software is much easier to debug because it allows you to pinpoint precisely exactly where and when a difficulty happens.
Begin with unit assessments, which target particular person capabilities or modules. These compact, isolated checks can immediately expose whether a specific piece of logic is working as envisioned. Any time a exam fails, you straight away know where by to glance, appreciably cutting down enough time put in debugging. Unit exams are Specifically helpful for catching regression bugs—issues that reappear just after Beforehand currently being set.
Subsequent, combine integration checks and conclude-to-stop tests into your workflow. These assistance be sure that a variety of elements of your application do the job alongside one another efficiently. They’re notably beneficial for catching bugs that happen in complex units with a number of elements or solutions interacting. If something breaks, your assessments can let you know which Element of the pipeline unsuccessful and under what disorders.
Composing tests also forces you to definitely Believe critically regarding your code. To test a aspect adequately, you will need to understand its inputs, predicted outputs, and edge cases. This standard of understanding Obviously prospects to raised code structure and less bugs.
When debugging a difficulty, composing a failing exam that reproduces the bug may be a robust first step. When the exam fails constantly, you could concentrate on repairing the bug and check out your check move when The difficulty is settled. This tactic ensures that precisely the same bug doesn’t return Down the road.
In brief, producing checks turns debugging from a aggravating guessing video game right into a structured and predictable course of action—assisting you catch additional bugs, a lot quicker and much more reliably.
Get Breaks
When debugging a tough difficulty, it’s simple to become immersed in the challenge—gazing your monitor for several hours, trying Remedy soon after Option. But one of the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lower annoyance, and infrequently see The difficulty from the new point of view.
If you're far too near the code for much too long, cognitive exhaustion sets in. You might start overlooking noticeable errors or misreading code that you wrote just hours before. In this point out, your Mind becomes much less effective at issue-solving. A short wander, a espresso crack, or simply switching to another undertaking for ten–15 minutes can refresh your focus. Many builders report obtaining the basis of an issue when they've taken time and energy to disconnect, allowing their subconscious perform inside the track record.
Breaks also help reduce burnout, Primarily through extended debugging periods. Sitting down in front of a screen, mentally trapped, is don't just unproductive and also draining. Stepping away enables you to return with renewed energy in addition to a clearer way of thinking. You could possibly abruptly recognize a missing semicolon, a logic flaw, or perhaps a misplaced variable that eluded you before.
For those who’re caught, a great general guideline should be to established a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver all-around, stretch, or do one thing unrelated to code. It may well truly feel counterintuitive, especially beneath tight deadlines, nonetheless it really brings about quicker and more practical debugging in the long run.
In a nutshell, having breaks just isn't an indication of weakness—it’s a smart tactic. It gives your brain Place to breathe, improves your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Understand From Each individual Bug
Each bug you face is a lot more than just A brief setback—It is really an opportunity to expand being a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can educate you a thing important if you take some time to mirror and assess what went Completely wrong.
Start by asking your self several essential issues when the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught earlier with better practices like unit testing, code reviews, or logging? The responses often expose blind places as part of your workflow or being familiar with and help you build stronger coding habits moving ahead.
Documenting bugs will also be a wonderful pattern. Retain a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and Everything you discovered. Over time, you’ll begin to see designs—recurring concerns or frequent errors—that you can proactively avoid.
In workforce environments, sharing That which you've realized from a bug with your friends might be Specifically potent. Whether it’s via a Slack concept, a brief generate-up, or A fast information-sharing session, assisting Many others stay away from the exact same difficulty boosts staff efficiency and cultivates a much better Finding out culture.
Additional importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. Rather than dreading bugs, you’ll get started appreciating them as vital elements of your enhancement journey. In fact, a lot of the ideal developers are not those who compose perfect code, but people that constantly master from their problems.
Ultimately, Just about every bug you resolve provides a brand new layer on your skill established. So next time you squash a bug, take a minute to reflect—you’ll arrive absent a smarter, much more capable developer thanks to it.
Conclusion
Strengthening your debugging skills will take time, exercise, and patience — nevertheless the payoff is large. It makes you a more successful, self-assured, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be improved at Everything you do.